Contents [hide ]
Many to Many Mapping
Many To Many mapping to represent a many to many relationships between tables. A many to many relationships occurs when many records from one table correspond to many records from another table.
For example, many persons can have many hobbies and a hobby can belong to many persons. So many records from the person table can correspond to many records from the hobby table.
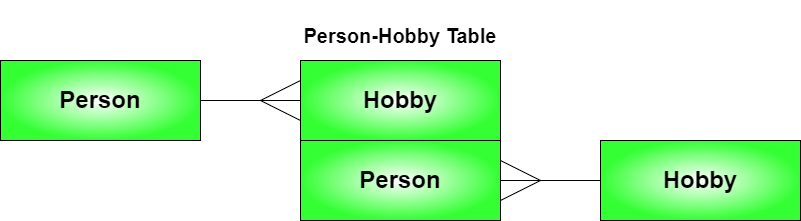
Tools and Technologies Used:
1. Java 8
2. MySQL 8
3. Eclipse IDE
4. mysql-connector-java-8.0.23.jar
5. Hibernate 5.5.7.Final
6. Maven 3.6.1
Maven Project for Many to Many Mapping
We need to follow below steps:
Step 1: Click on File --> New --> Maven Project.
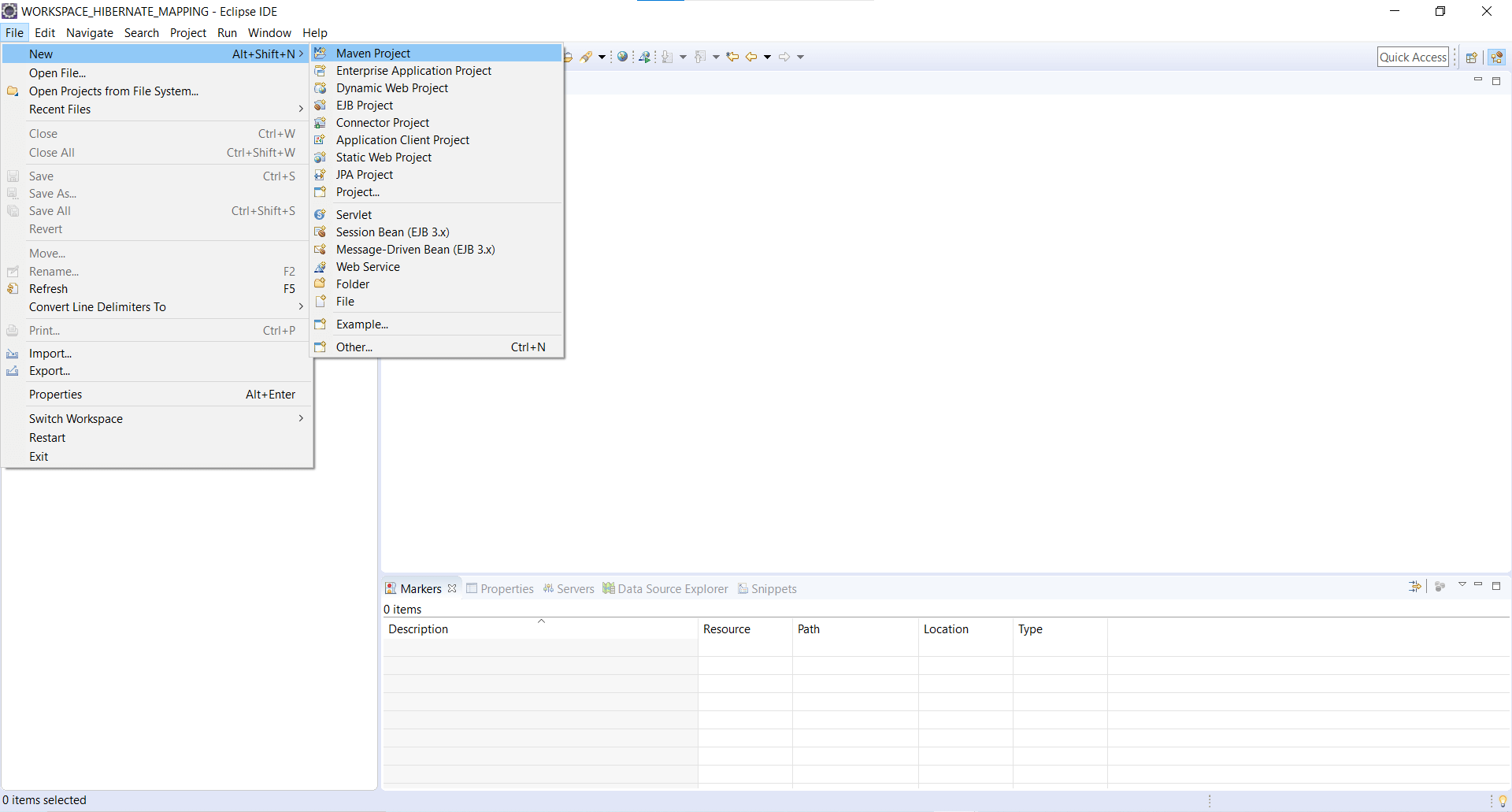
Step 2: Check Checkbox Create a simple project (skip archetype selection). Click on Next button.
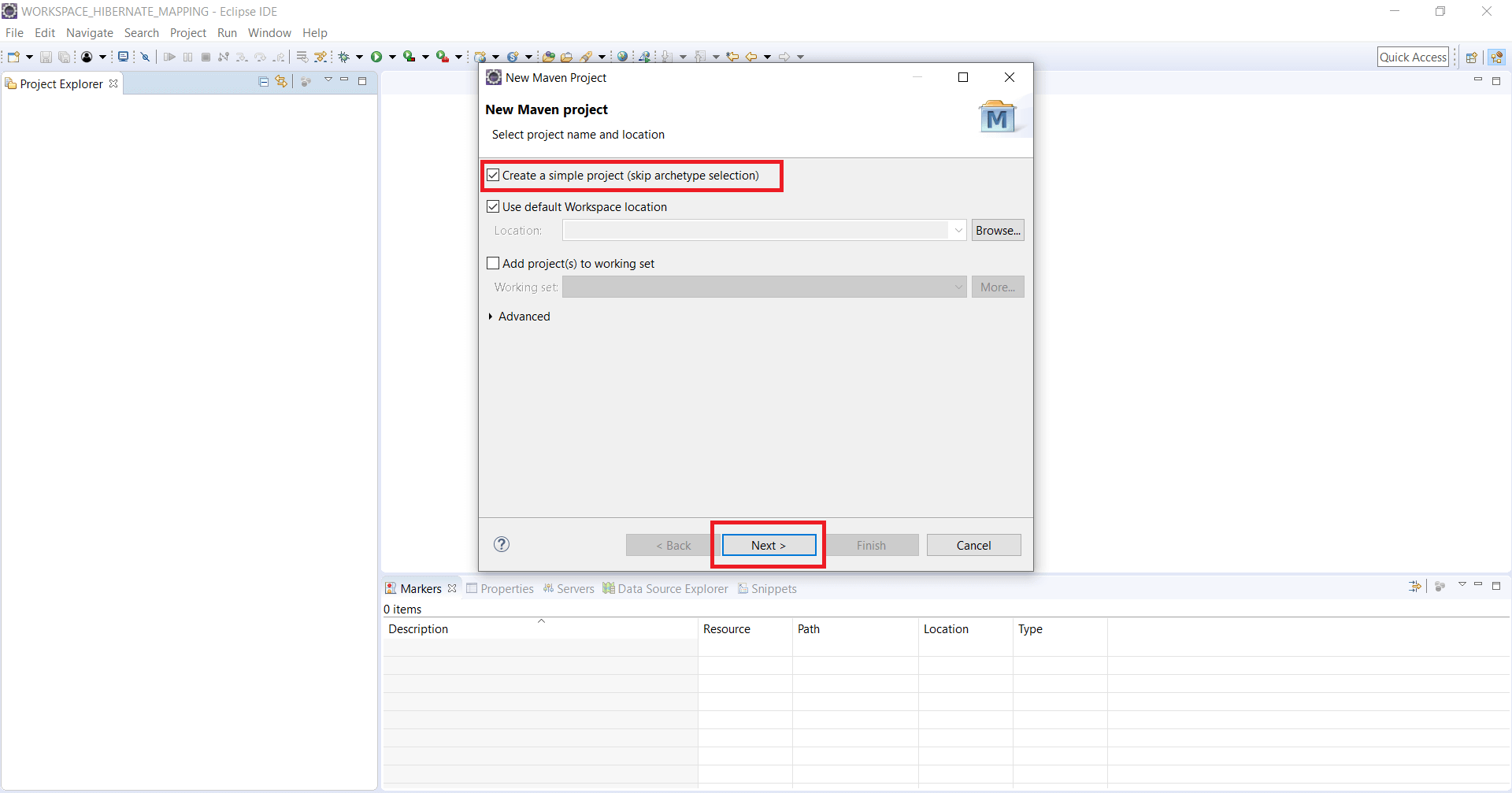
Step 3: Enter Group Id, Artifact Id, Version, Packaging, Name, Description details and click on Finish button.
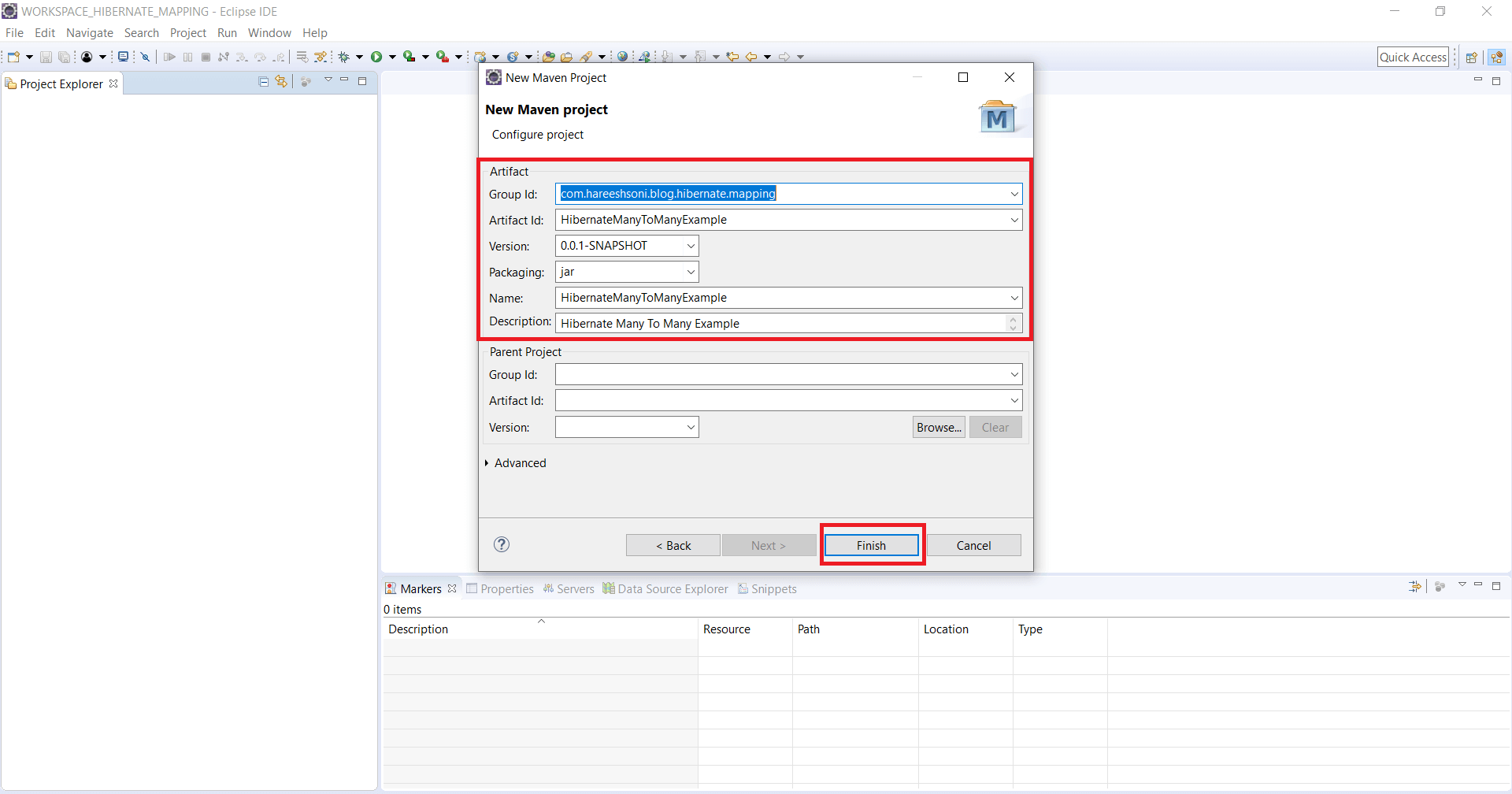
Update pom.xml file.
Open pom.xml file and paste below pom.xml file code.
4.0.0 com.hareeshsoni.blog.hibernate.mapping HibernateManyToManyExample 0.0.1-SNAPSHOT HibernateManyToManyExample Hibernate Many To Many Example org.hibernate hibernate-core 5.5.7.Final mysql mysql-connector-java 8.0.23 org.apache.maven.plugins maven-compiler-plugin 3.6.1 1.8 1.8
Update Maven Project
Step 1: Right-click on project HibernateManyToManyExample --> Maven --> Update Project…
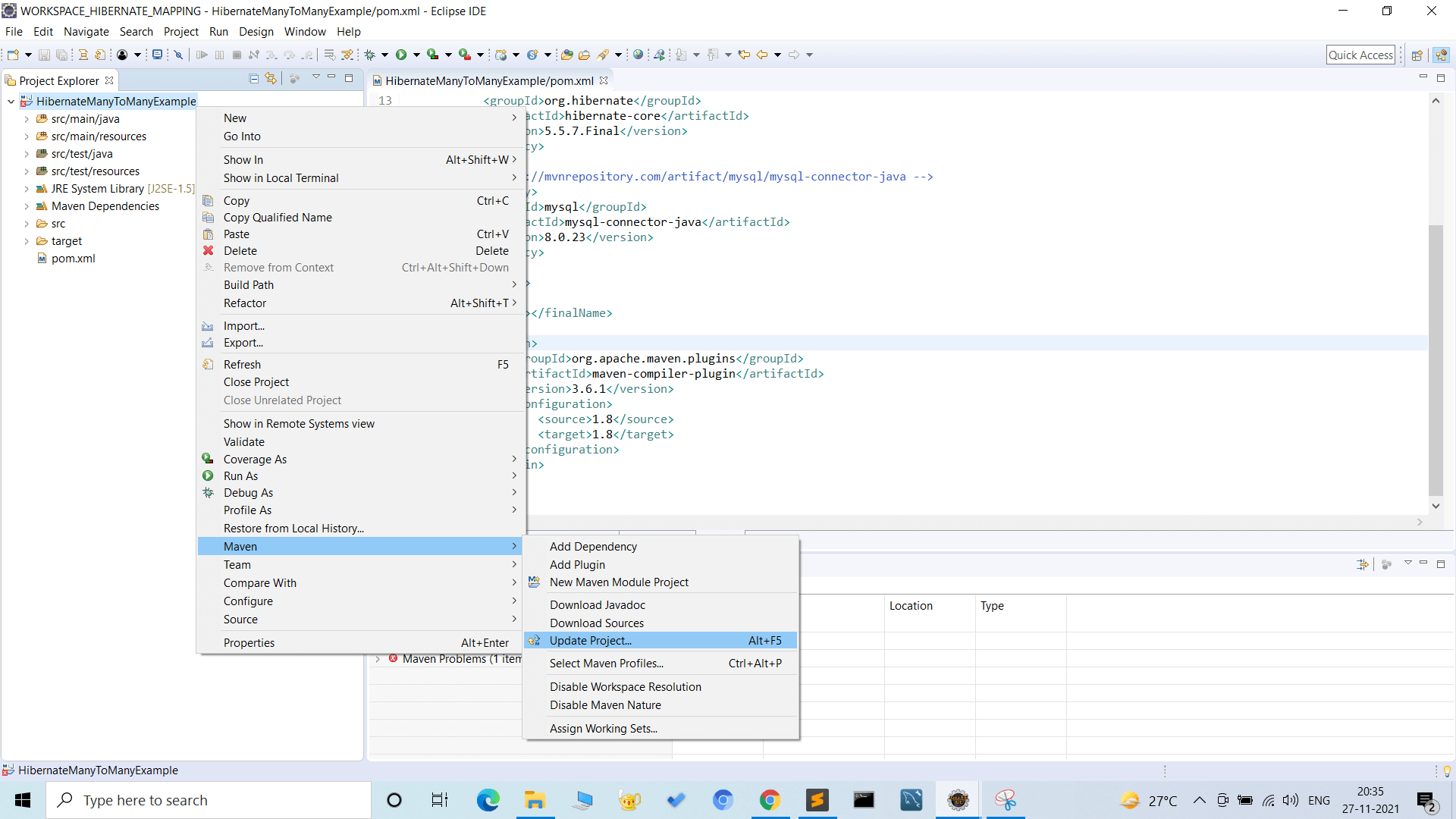
Step 2: Check Checkbox Force Update of Snapshots/Releases. Click on OK Button.
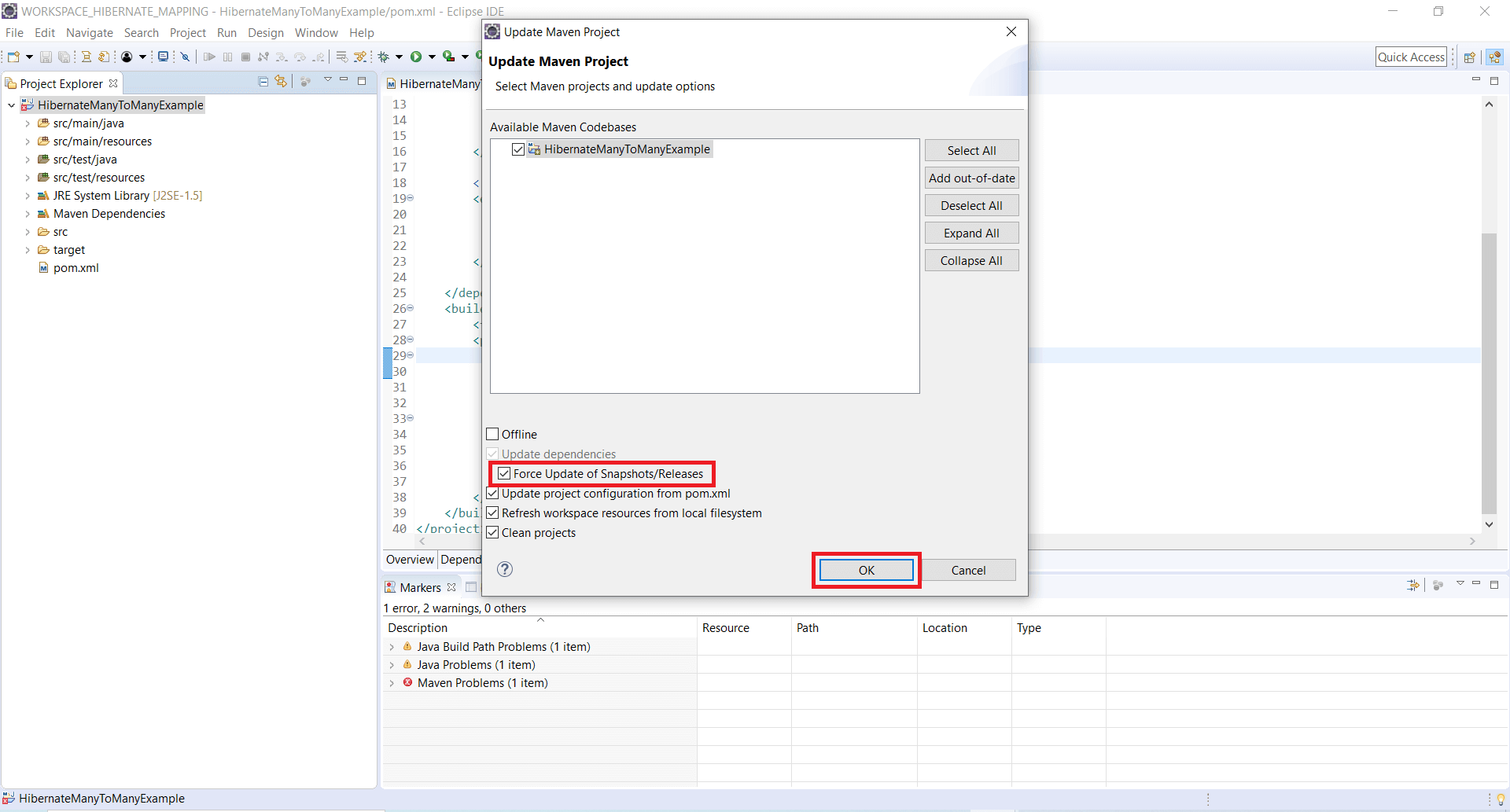
We have used the below JPA Annotations:
@Entity: @Entity annotation specifies that the class is an entity.
@Table: @Table annotation specifies the primary table for the annotated entity.
@Id: @Id annotation marks the particular field as the primary key of the Entity.
@GeneratedValue: This annotation is used to specify how the primary key should be generated.
@Column: This annotation maps the corresponding fields to their respective columns in the database table.
@JoinColumn: This annotation defines the foreign key. It is the column that associates the two tables.
@ManyToMany: This annotation is used to create many to many relationship between Person and Hobby entities.
@JoinTable: This annotation is used to define the join table (link table) for many-to-many relationship.
package com.hareeshsoni.blog.hibernate.mapping.model; import java.util.HashSet; import java.util.Set; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.ManyToMany; import javax.persistence.Table; @Entity @Table(name = "hobby") public class Hobby { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @Column(name = "hobby_id") private int hobbyId; @Column(name = "hobby_name") private String hobbyName; @ManyToMany(mappedBy = "hobbies") private Setpersons = new HashSet (); public Hobby() { } public Hobby(String hobbyName) { this.hobbyName = hobbyName; } public int getHobbyId() { return hobbyId; } public void setHobbyId(int hobbyId) { this.hobbyId = hobbyId; } public String getHobbyName() { return hobbyName; } public void setHobbyName(String hobbyName) { this.hobbyName = hobbyName; } public Set getPersons() { return persons; } public void setPersons(Set persons) { this.persons = persons; } }
package com.hareeshsoni.blog.hibernate.mapping.model; import java.util.HashSet; import java.util.Set; import javax.persistence.CascadeType; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.JoinColumn; import javax.persistence.JoinTable; import javax.persistence.ManyToMany; import javax.persistence.Table; @Entity @Table(name = "person") public class Person { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @Column(name = "person_id") private int personId; @Column(name = "person_name") private String personName; @ManyToMany(cascade = { CascadeType.ALL }) @JoinTable(name = "person_hobby", joinColumns = { @JoinColumn(name = "person_id") }, inverseJoinColumns = { @JoinColumn(name = "hobby_id") }) private Sethobbies = new HashSet (); public Person() { } public Person(String personName) { this.personName = personName; } public int getPersonId() { return personId; } public void setPersonId(int personId) { this.personId = personId; } public String getPersonName() { return personName; } public void setPersonName(String personName) { this.personName = personName; } public Set getHobbies() { return hobbies; } public void setHobbies(Set hobbies) { this.hobbies = hobbies; } }
package com.hareeshsoni.blog.hibernate.mapping.util; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; public class HibernateUtil { private static SessionFactory sessionFactory; private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure("hibernate.cfg.xml"); System.out.println("Hibernate configuration file loaded"); SessionFactory sessionFactory = configuration.buildSessionFactory(); return sessionFactory; } public static SessionFactory getSessionFactory() { if(sessionFactory == null) sessionFactory = buildSessionFactory(); return sessionFactory; } }
In hibernate.cfg.xml file provide correct username and password.
Create database name "many_to_many_mapping" inside mysql database.
com.mysql.cj.jdbc.Driver jdbc:mysql://localhost:3306/many_to_many_mapping root ######## org.hibernate.dialect.MySQL5Dialect true update
package com.hareeshsoni.blog.hibernate.mapping; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.hareeshsoni.blog.hibernate.mapping.model.Hobby; import com.hareeshsoni.blog.hibernate.mapping.model.Person; import com.hareeshsoni.blog.hibernate.mapping.util.HibernateUtil; public class MainApp { public static void main(String[] args) { SessionFactory sessionFactory = HibernateUtil.getSessionFactory(); Session session = sessionFactory.openSession(); session.beginTransaction(); Person person1 = new Person("Mohan"); Person person2 = new Person("Sohan"); Hobby hobby1 = new Hobby("Reading"); Hobby hobby2 = new Hobby("Painting"); person1.getHobbies().add(hobby1); person1.getHobbies().add(hobby2); person2.getHobbies().add(hobby2); session.save(person1); session.save(person2); session.getTransaction().commit(); session.close(); sessionFactory.close(); } }
Now run MainApp.java
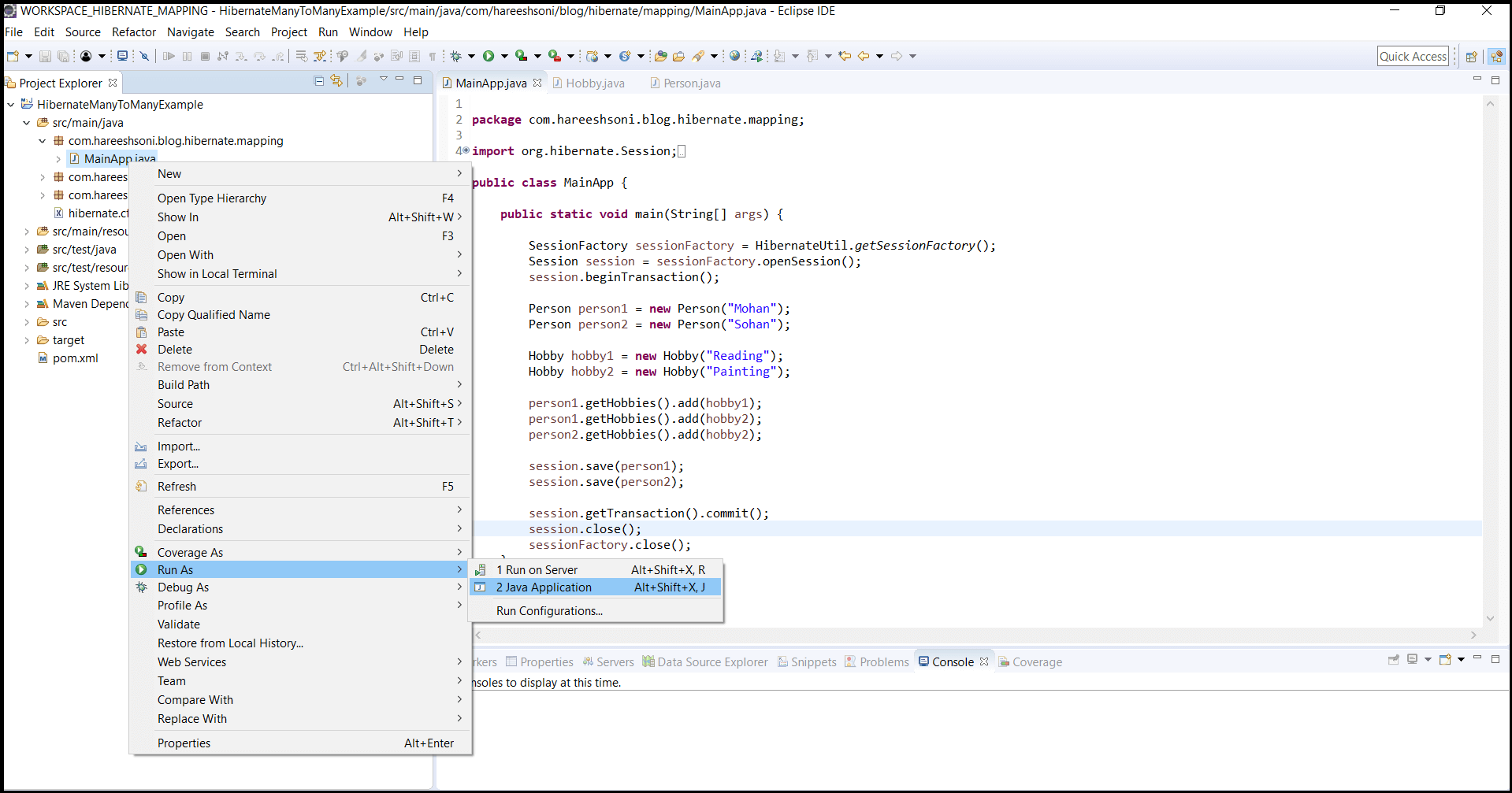
Output
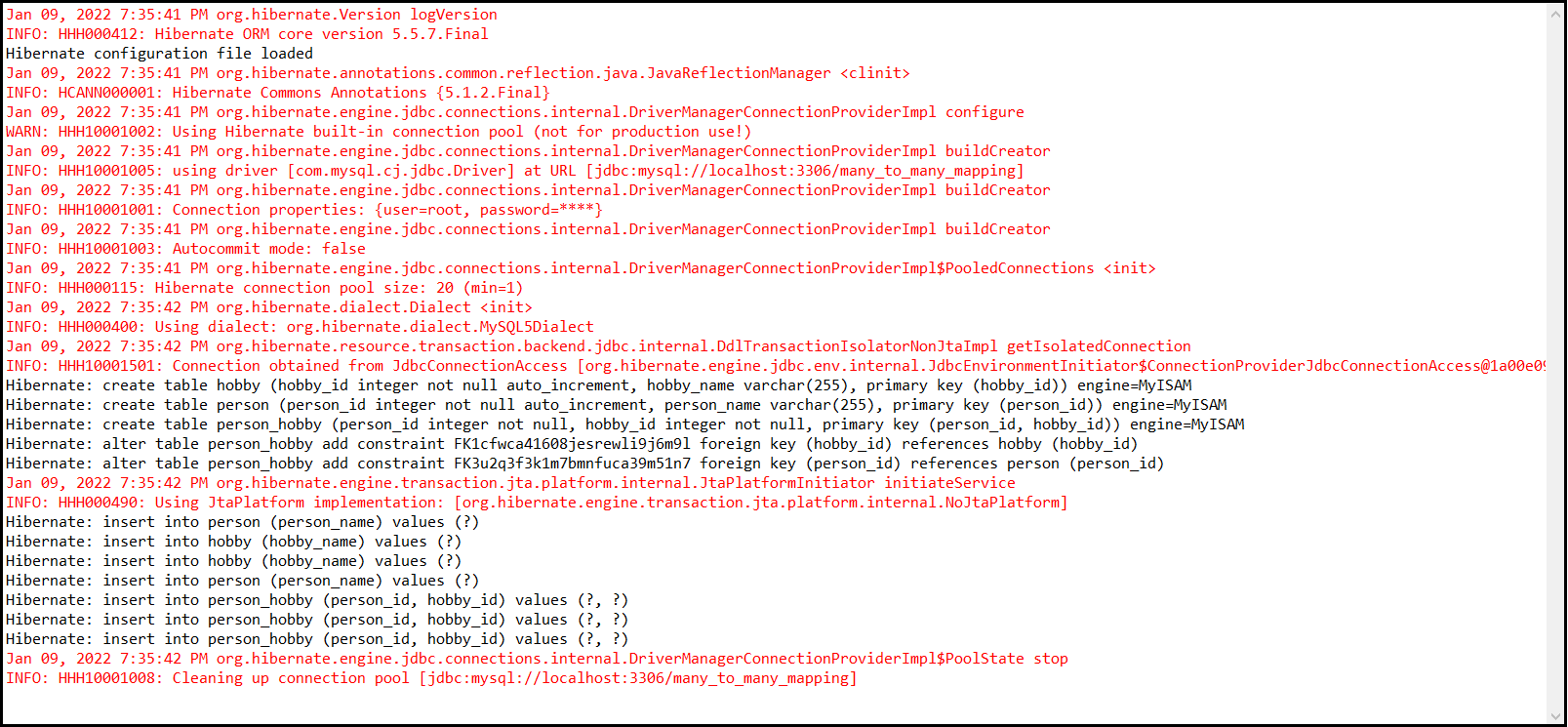
Database tables:
Person Table
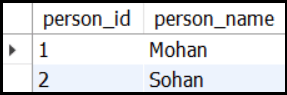
Hobby Table
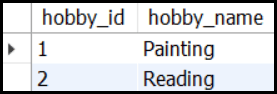
Person-Hobby Table
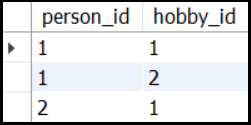