Contents [hide ]
One to One Mapping
One To One mapping to represent a one to one relationship between database tables. A one to one relationship occurs between tables when one record from the first table corresponds to only one record in the second table.
For example, an Account can belong to one person. So one record in an Account table will correspond to only one record in the Person table.

Tools and Technologies Used:
1. Java 8
2. MySQL 8
3. Eclipse IDE
4. mysql-connector-java-8.0.23.jar
5. Hibernate 5.5.7.Final
6. Maven 3.6.1
Maven Project for One to One Mapping
We need to follow below steps:
Step 1: Click on File --> New --> Maven Project.
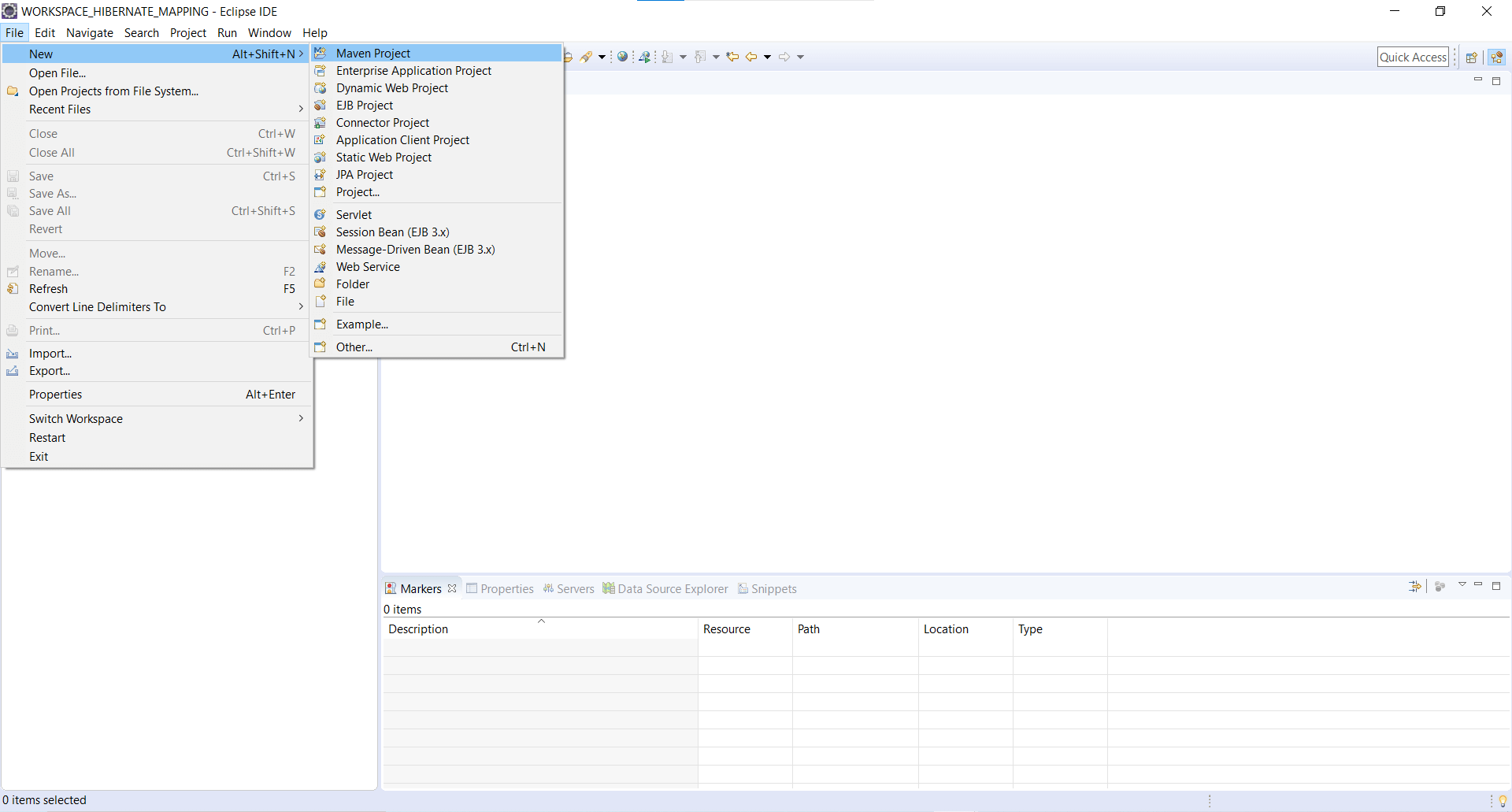
Step 2: Check Checkbox Create a simple project (skip archetype selection). Click on Next button.
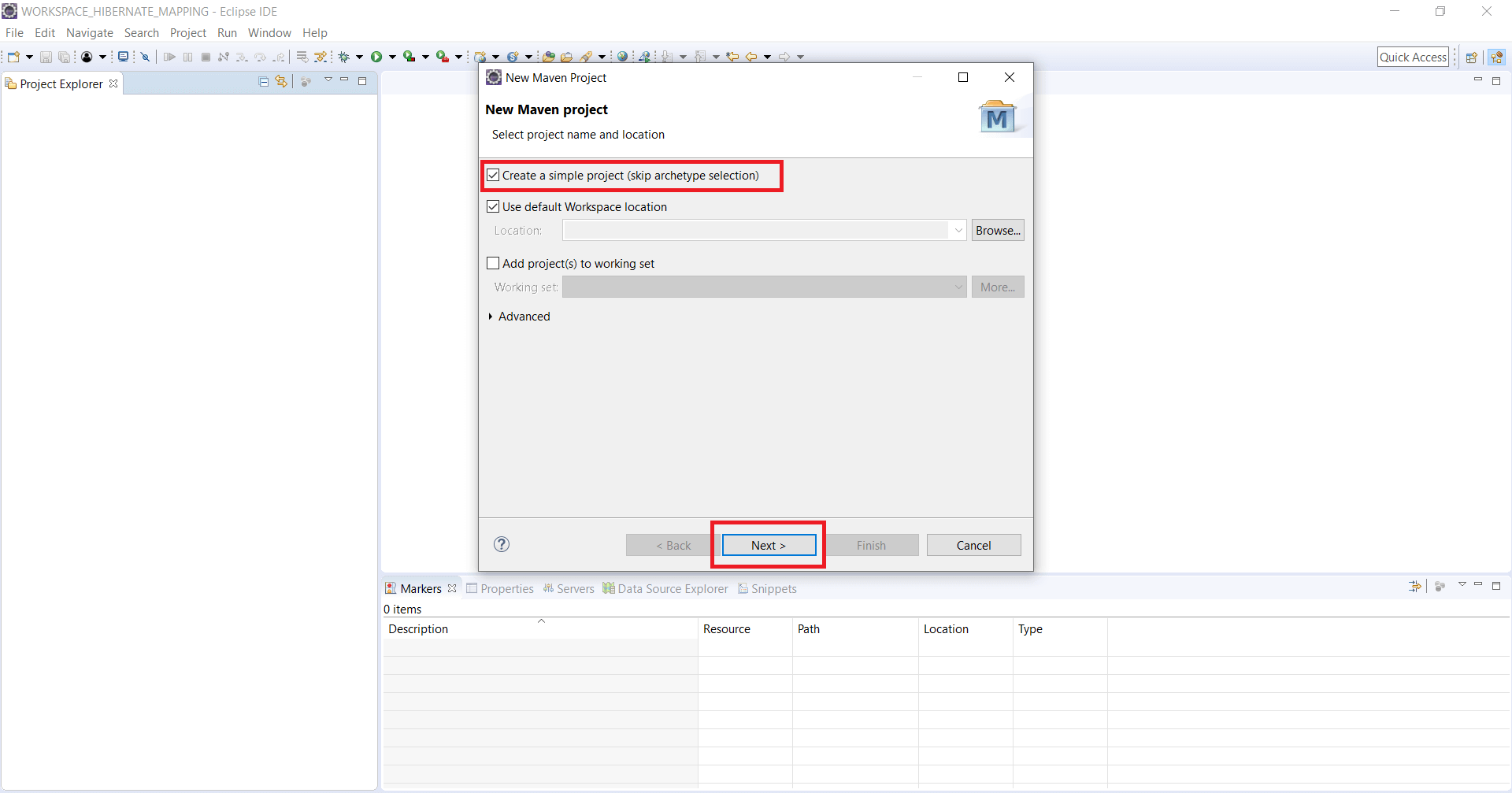
Step 3: Enter Group Id, Artifact Id, Version, Packaging, Name, Description details and click on Finish button.
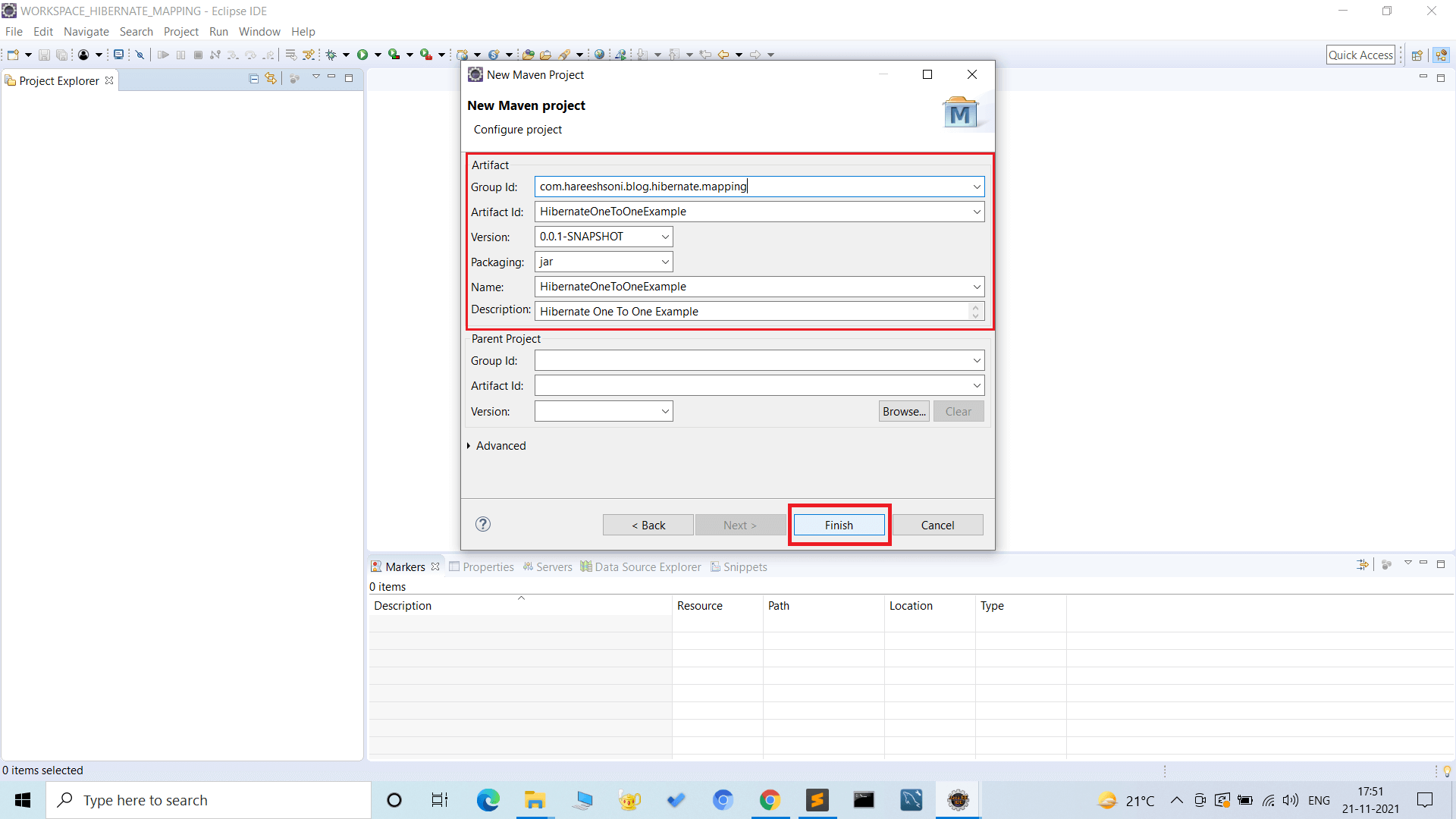
Update pom.xml file.
Open pom.xml file and paste below pom.xml file code.
4.0.0 com.hareeshsoni.blog.hibernate.mapping HibernateOneToOneExample 0.0.1-SNAPSHOT HibernateOneToOneExample Hibernate One To One Example org.hibernate hibernate-core 5.5.7.Final mysql mysql-connector-java 8.0.23 org.apache.maven.plugins maven-compiler-plugin 3.6.1 1.8 1.8
Update Maven Project
Step 1: Right-click on project HibernateOneToOneExample --> Maven --> Update Project…
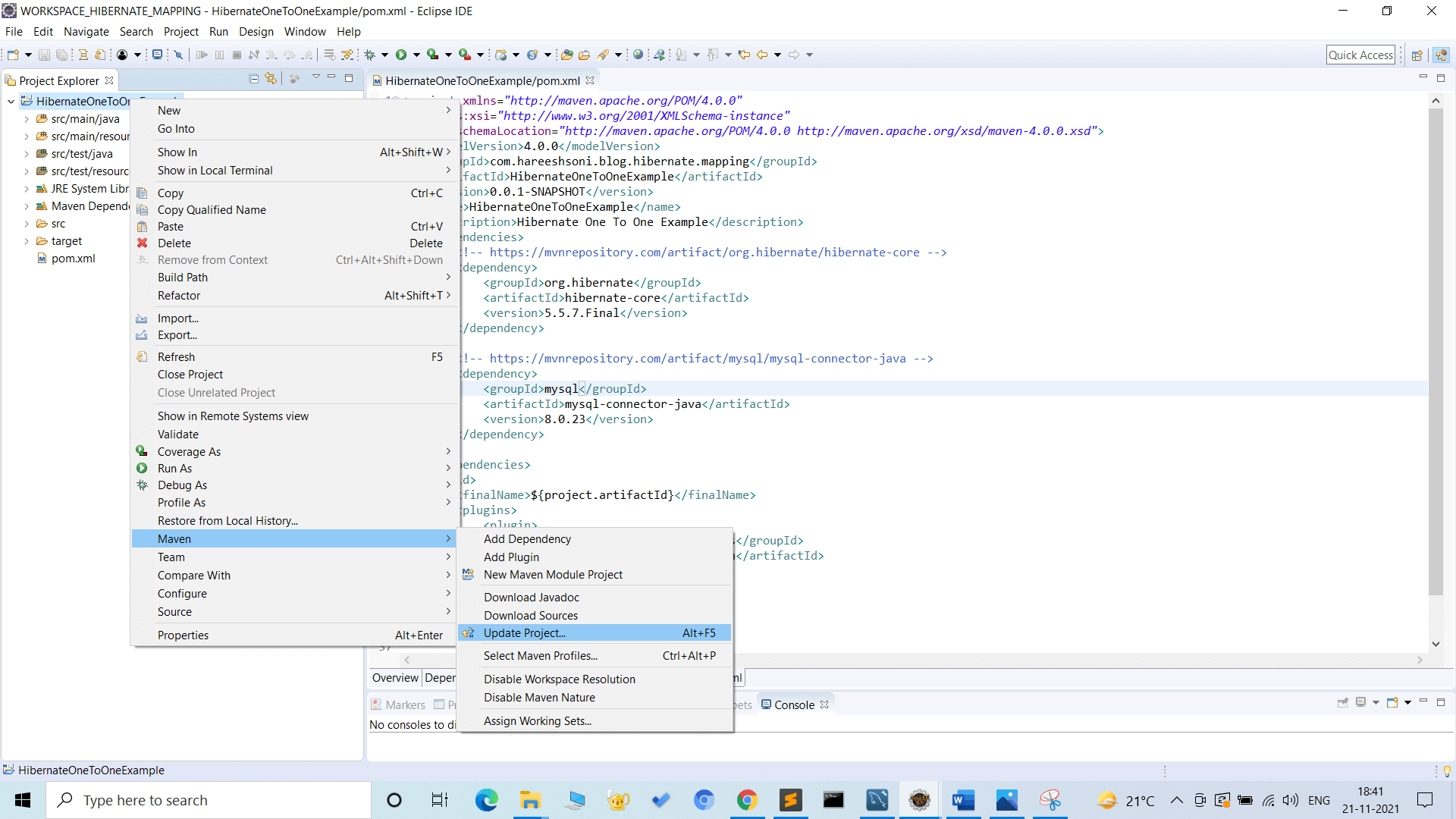
Step 2: Check Checkbox Force Update of Snapshots/Releases. Click on OK Button.
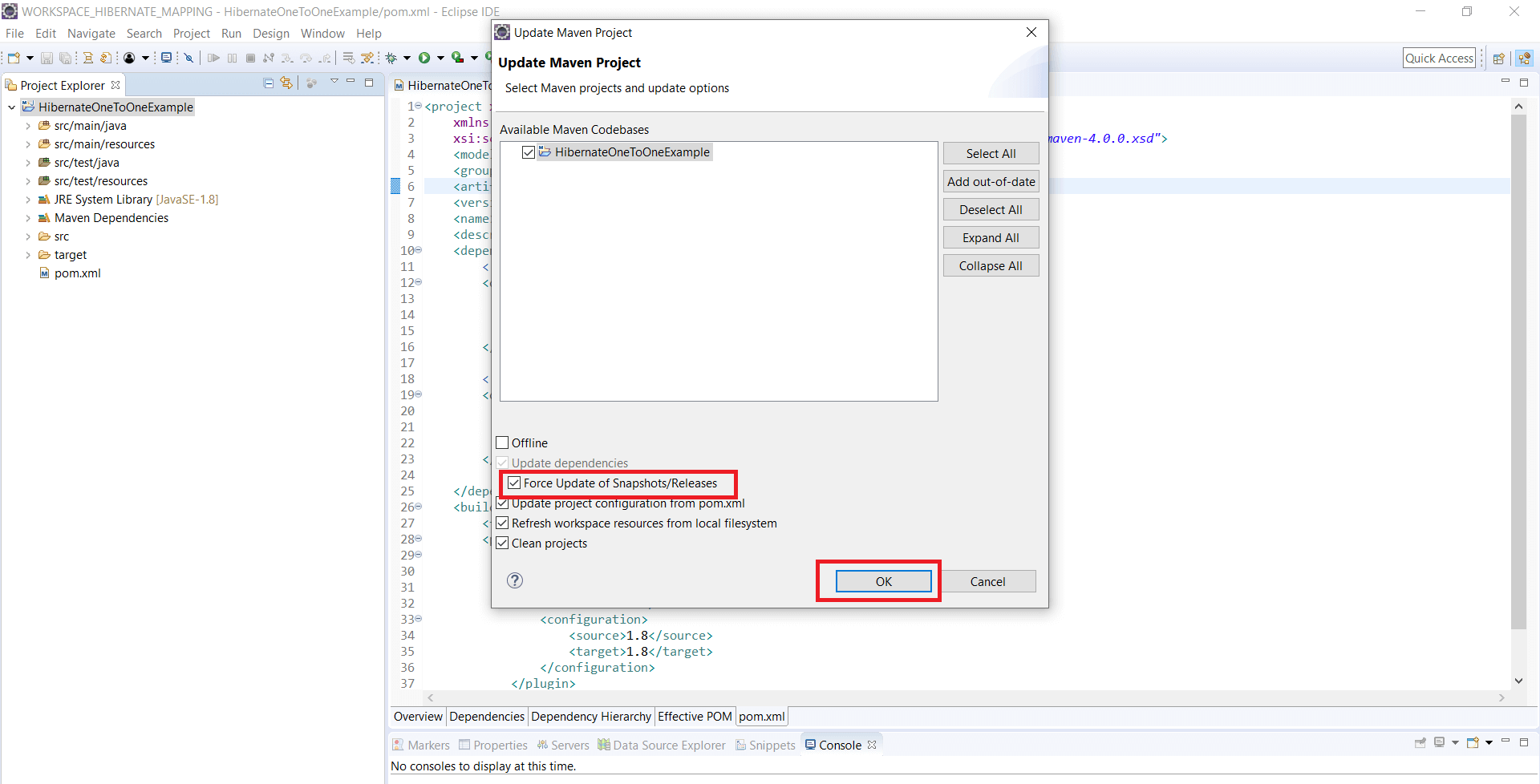
We have used the below JPA Annotations:
@Entity: @Entity annotation specifies that the class is an entity.
@Table: @Table annotation specifies the primary table for the annotated entity.
@Id: @Id annotation marks the particular field as the primary key of the Entity.
@GeneratedValue: This annotation is used to specify how the primary key should be generated.
@Column: This annotation maps the corresponding fields to their respective columns in the database table.
@JoinColumn: This annotation defines the foreign key. It is the column that associates the two tables.
@OneToOne: This annotation is used to create one to one relationship between Person and Account entities.
package com.hareeshsoni.blog.hibernate.mapping.model; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name = "account") public class Account { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @Column(name = "account_id") private int accountId; @Column(name = "account_number") private String accountNumber; public Account() { } public Account(String accountNumber) { this.accountNumber = accountNumber; } public int getAccountId() { return accountId; } public void setAccountId(int accountId) { this.accountId = accountId; } public String getAccountNumber() { return accountNumber; } public void setAccountNumber(String accountNumber) { this.accountNumber = accountNumber; } }
package com.hareeshsoni.blog.hibernate.mapping.model; import javax.persistence.CascadeType; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.JoinColumn; import javax.persistence.OneToOne; import javax.persistence.Table; @Entity @Table(name = "person") public class Person { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @Column(name = "person_id") private int personId; @Column(name = "person_name") private String personName; @OneToOne(cascade = CascadeType.ALL) @JoinColumn(name = "account_id") private Account account; public Person() { } public Person(String personName) { this.personName = personName; } public int getPersonId() { return personId; } public void setPersonId(int personId) { this.personId = personId; } public String getPersonName() { return personName; } public void setPersonName(String personName) { this.personName = personName; } public Account getAccount() { return account; } public void setAccount(Account account) { this.account = account; } }
package com.hareeshsoni.blog.hibernate.mapping.util; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; public class HibernateUtil { private static SessionFactory sessionFactory; private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure("hibernate.cfg.xml"); System.out.println("Hibernate configuration file loaded"); SessionFactory sessionFactory = configuration.buildSessionFactory(); return sessionFactory; } public static SessionFactory getSessionFactory() { if(sessionFactory == null) sessionFactory = buildSessionFactory(); return sessionFactory; } }
In hibernate.cfg.xml file provide correct username and password.
Create database name "one_to_one_mapping" inside mysql database.
com.mysql.cj.jdbc.Driver jdbc:mysql://localhost:3306/one_to_one_mapping root ######## org.hibernate.dialect.MySQL5Dialect true update
package com.hareeshsoni.blog.hibernate.mapping; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.hareeshsoni.blog.hibernate.mapping.model.Account; import com.hareeshsoni.blog.hibernate.mapping.model.Person; import com.hareeshsoni.blog.hibernate.mapping.util.HibernateUtil; public class MainApp { public static void main(String[] args) { SessionFactory sessionFactory = HibernateUtil.getSessionFactory(); Session session = sessionFactory.openSession(); session.beginTransaction(); Account account = new Account(); account.setAccountNumber("ACC-1001"); Person person = new Person(); person.setPersonName("Mohan"); person.setAccount(account); session.save(person); Account newAccount = new Account(); newAccount.setAccountNumber("ACC-1002"); Person newPerson = new Person(); newPerson.setPersonName("Sohan"); newPerson.setAccount(newAccount); session.save(newPerson); session.getTransaction().commit(); session.close(); sessionFactory.close(); } }
Now run MainApp.java
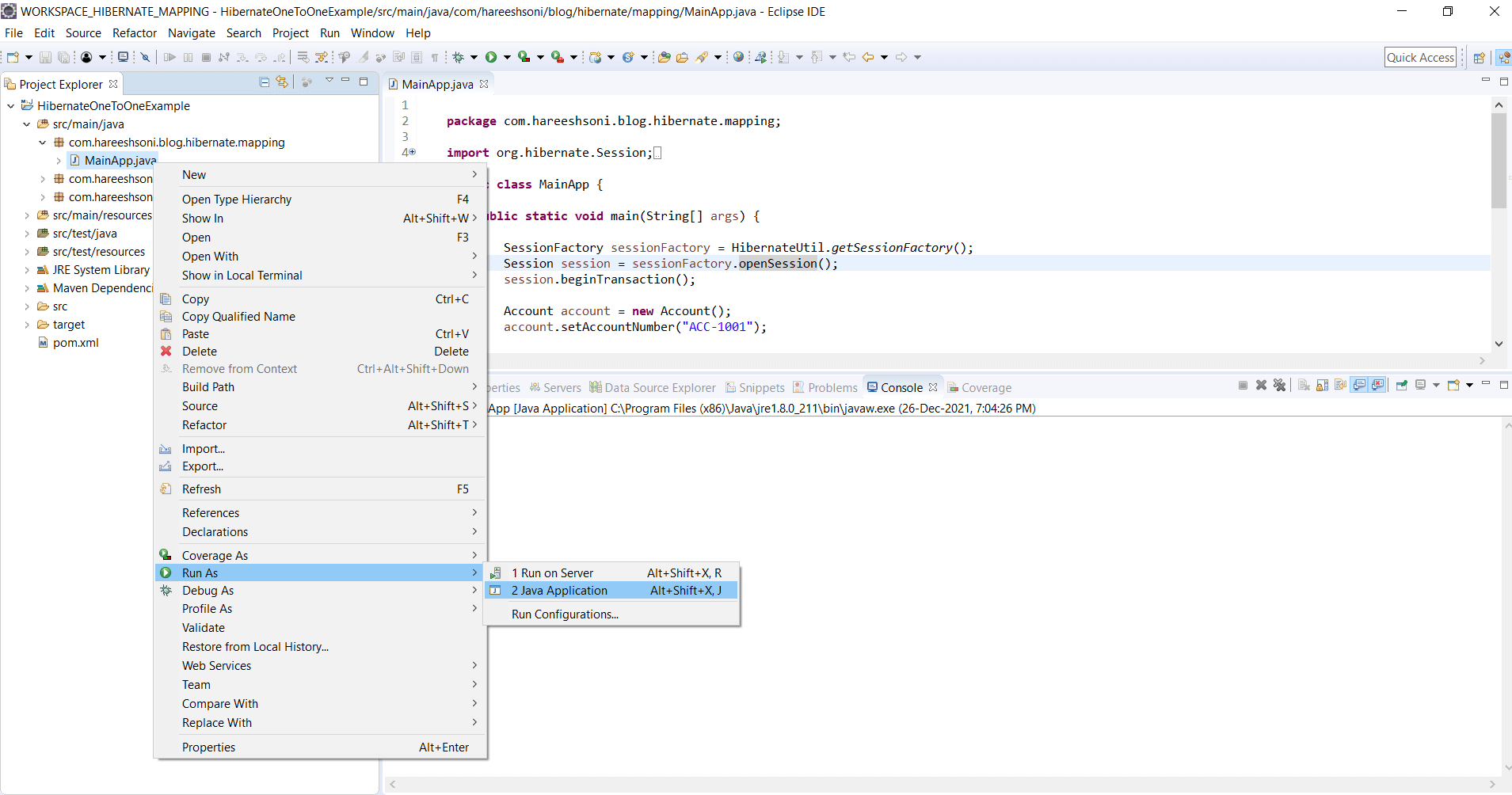
Output
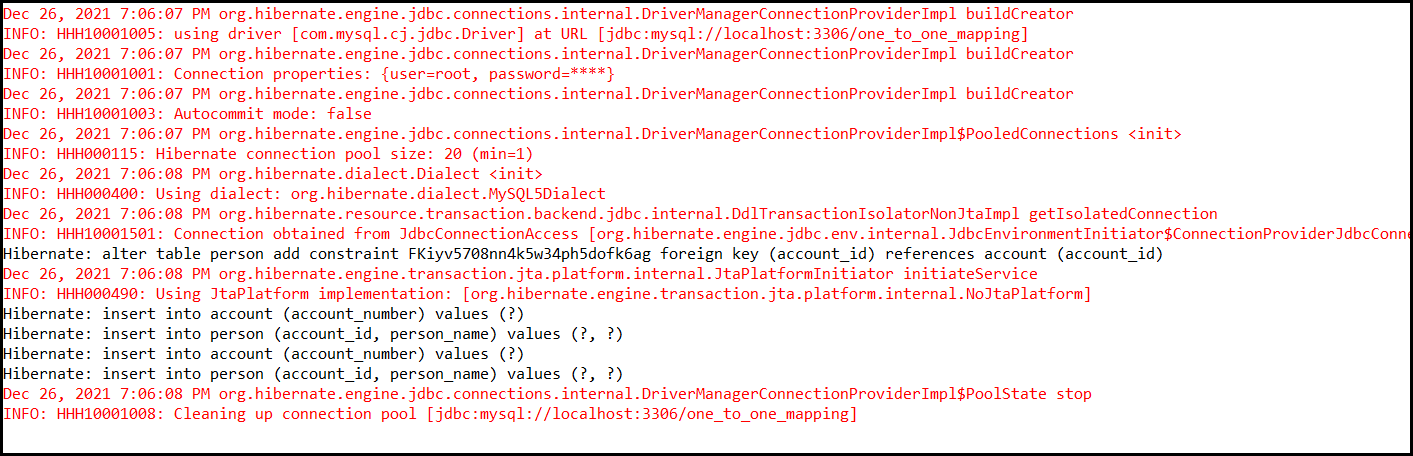
Database tables:
Account Table
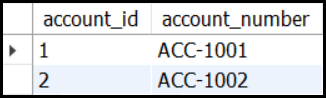
Person Table
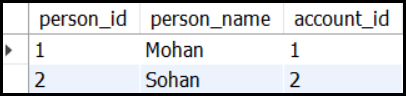